在进行WPF界面设计时,我们需要在很多地方设置颜色属性,比如元素的背景色、前景色以及边框的颜色,还有形状的内部填充和笔画,这些颜色的设置在WPF中都以画刷(Brush)的形式实现。比如最常用的画刷就是SolidColorBrush,它表示一种纯色。
画刷被定义成一个抽象类,如下所示。
public abstract class Brush : Animatable, IFormattable, IResource
{
public static readonly DependencyProperty OpacityProperty;
public static readonly DependencyProperty TransformProperty;
public static readonly DependencyProperty RelativeTransformProperty;
protected Brush();
public double Opacity { get; set; }
public Transform Transform { get; set; }
public Transform RelativeTransform { get; set; }
public Brush Clone();
public Brush CloneCurrentValue();
public override string ToString();
public string ToString(IFormatProvider provider);
}
Brush继承于Animatable基类和Freezable基类,说明它支持更改通知。如果改变了画刷,任何使用该画刷的控件的颜色都会自动重绘。
Opacity 属性表示它支持部分透明。
Brush一共有7个子类,下表中列出了它们的信息。
画刷名称 | 功能说明 |
SolidColorBrush | 使用单一的连续颜色填充区域 |
LinearGradientBrush | 使用线性渐变绘制区域。 |
RadialGradientBrush | 使用径向渐变绘制区域。 焦点定义渐变的开始,而圆定义渐变的终点。 |
ImageBrush | 使用图像绘制区域。 |
VisualBrush | 使用一个视图绘制区域。 |
BitmapCacheBrush | 绘制带有缓存的内容的区域。 |
DrawingBrush | 使用包括形状、文本、视频、图像或其他绘制项等填充区域。 |
关于Brush基类和子类的继承关系,参考下图所示。
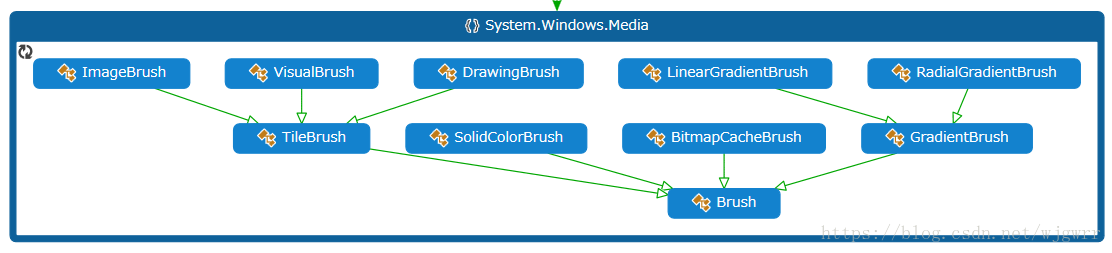
另外,WPF还定义了一些默认画刷,这些画刷都被定义在Brushes类中。如下所示
public sealed class Brushes
{
public static SolidColorBrush AliceBlue { get; }
public static SolidColorBrush PaleGoldenrod { get; }
public static SolidColorBrush Orchid { get; }
public static SolidColorBrush OrangeRed { get; }
public static SolidColorBrush Orange { get; }
public static SolidColorBrush OliveDrab { get; }
public static SolidColorBrush Olive { get; }
public static SolidColorBrush OldLace { get; }
public static SolidColorBrush Navy { get; }
public static SolidColorBrush NavajoWhite { get; }
public static SolidColorBrush Moccasin { get; }
public static SolidColorBrush MistyRose { get; }
public static SolidColorBrush MintCream { get; }
public static SolidColorBrush MidnightBlue { get; }
public static SolidColorBrush MediumVioletRed { get; }
public static SolidColorBrush MediumTurquoise { get; }
public static SolidColorBrush MediumSpringGreen { get; }
public static SolidColorBrush MediumSlateBlue { get; }
public static SolidColorBrush LightSkyBlue { get; }
public static SolidColorBrush LightSlateGray { get; }
public static SolidColorBrush LightSteelBlue { get; }
public static SolidColorBrush LightYellow { get; }
public static SolidColorBrush Lime { get; }
public static SolidColorBrush LimeGreen { get; }
public static SolidColorBrush PaleGreen { get; }
public static SolidColorBrush Linen { get; }
public static SolidColorBrush Maroon { get; }
public static SolidColorBrush MediumAquamarine { get; }
public static SolidColorBrush MediumBlue { get; }
public static SolidColorBrush MediumOrchid { get; }
public static SolidColorBrush MediumPurple { get; }
public static SolidColorBrush MediumSeaGreen { get; }
public static SolidColorBrush Magenta { get; }
public static SolidColorBrush PaleTurquoise { get; }
public static SolidColorBrush PaleVioletRed { get; }
public static SolidColorBrush PapayaWhip { get; }
public static SolidColorBrush SlateGray { get; }
public static SolidColorBrush Snow { get; }
public static SolidColorBrush SpringGreen { get; }
public static SolidColorBrush SteelBlue { get; }
public static SolidColorBrush Tan { get; }
public static SolidColorBrush Teal { get; }
public static SolidColorBrush SlateBlue { get; }
public static SolidColorBrush Thistle { get; }
public static SolidColorBrush Transparent { get; }
public static SolidColorBrush Turquoise { get; }
public static SolidColorBrush Violet { get; }
public static SolidColorBrush Wheat { get; }
public static SolidColorBrush White { get; }
public static SolidColorBrush WhiteSmoke { get; }
public static SolidColorBrush Tomato { get; }
public static SolidColorBrush LightSeaGreen { get; }
public static SolidColorBrush SkyBlue { get; }
public static SolidColorBrush Sienna { get; }
public static SolidColorBrush PeachPuff { get; }
public static SolidColorBrush Peru { get; }
public static SolidColorBrush Pink { get; }
public static SolidColorBrush Plum { get; }
public static SolidColorBrush PowderBlue { get; }
public static SolidColorBrush Purple { get; }
public static SolidColorBrush Silver { get; }
public static SolidColorBrush Red { get; }
public static SolidColorBrush RoyalBlue { get; }
public static SolidColorBrush SaddleBrown { get; }
public static SolidColorBrush Salmon { get; }
public static SolidColorBrush SandyBrown { get; }
public static SolidColorBrush SeaGreen { get; }
public static SolidColorBrush SeaShell { get; }
public static SolidColorBrush RosyBrown { get; }
public static SolidColorBrush Yellow { get; }
public static SolidColorBrush LightSalmon { get; }
public static SolidColorBrush LightGreen { get; }
public static SolidColorBrush DarkRed { get; }
public static SolidColorBrush DarkOrchid { get; }
public static SolidColorBrush DarkOrange { get; }
public static SolidColorBrush DarkOliveGreen { get; }
public static SolidColorBrush DarkMagenta { get; }
public static SolidColorBrush DarkKhaki { get; }
public static SolidColorBrush DarkGreen { get; }
public static SolidColorBrush DarkGray { get; }
public static SolidColorBrush DarkGoldenrod { get; }
public static SolidColorBrush DarkCyan { get; }
public static SolidColorBrush DarkBlue { get; }
public static SolidColorBrush Cyan { get; }
public static SolidColorBrush Crimson { get; }
public static SolidColorBrush Cornsilk { get; }
public static SolidColorBrush CornflowerBlue { get; }
public static SolidColorBrush Coral { get; }
public static SolidColorBrush Chocolate { get; }
public static SolidColorBrush AntiqueWhite { get; }
public static SolidColorBrush Aqua { get; }
public static SolidColorBrush Aquamarine { get; }
public static SolidColorBrush Azure { get; }
public static SolidColorBrush Beige { get; }
public static SolidColorBrush Bisque { get; }
public static SolidColorBrush DarkSalmon { get; }
public static SolidColorBrush Black { get; }
public static SolidColorBrush Blue { get; }
public static SolidColorBrush BlueViolet { get; }
public static SolidColorBrush Brown { get; }
public static SolidColorBrush BurlyWood { get; }
public static SolidColorBrush CadetBlue { get; }
public static SolidColorBrush Chartreuse { get; }
public static SolidColorBrush BlanchedAlmond { get; }
public static SolidColorBrush DarkSeaGreen { get; }
public static SolidColorBrush DarkSlateBlue { get; }
public static SolidColorBrush DarkSlateGray { get; }
public static SolidColorBrush HotPink { get; }
public static SolidColorBrush IndianRed { get; }
public static SolidColorBrush Indigo { get; }
public static SolidColorBrush Ivory { get; }
public static SolidColorBrush Khaki { get; }
public static SolidColorBrush Lavender { get; }
public static SolidColorBrush Honeydew { get; }
public static SolidColorBrush LavenderBlush { get; }
public static SolidColorBrush LemonChiffon { get; }
public static SolidColorBrush LightBlue { get; }
public static SolidColorBrush LightCoral { get; }
public static SolidColorBrush LightCyan { get; }
public static SolidColorBrush LightGoldenrodYellow { get; }
public static SolidColorBrush LightGray { get; }
public static SolidColorBrush LawnGreen { get; }
public static SolidColorBrush LightPink { get; }
public static SolidColorBrush GreenYellow { get; }
public static SolidColorBrush Gray { get; }
public static SolidColorBrush DarkTurquoise { get; }
public static SolidColorBrush DarkViolet { get; }
public static SolidColorBrush DeepPink { get; }
public static SolidColorBrush DeepSkyBlue { get; }
public static SolidColorBrush DimGray { get; }
public static SolidColorBrush DodgerBlue { get; }
public static SolidColorBrush Green { get; }
public static SolidColorBrush Firebrick { get; }
public static SolidColorBrush ForestGreen { get; }
public static SolidColorBrush Fuchsia { get; }
public static SolidColorBrush Gainsboro { get; }
public static SolidColorBrush GhostWhite { get; }
public static SolidColorBrush Gold { get; }
public static SolidColorBrush Goldenrod { get; }
public static SolidColorBrush FloralWhite { get; }
public static SolidColorBrush YellowGreen { get; }
}
这些画刷都位于System.Windows.Media。
从下一节开始,我们就逐一讲解这些画刷的使用。
——重庆教主 2023年11月3日